Array:
An array? Array is a collection of variables of the same type. Let’s say you were to work with 4 different numbers as below:
int number1;
int number2;
int number3;
int number4;
So instead of creating these 4 numbers we can create one array of int type as below:
int[] numbers = new int[4];
First square bracket is to tell the compiler that we are declaring an array, second one is to set the size of the that array. In c# arrays have fix size and we have to specify during declaring it can not be changed.
Why use the keyword new?
In c# array is a class and when you are declaring an array, we are creating instance/object of array class.
To access the elements of an array we do it by giving the index of the array in square bracket, like code below.
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
In c# arrays are zero indexed which means that first element of the array has zero index. If you know the the value you will be storing in an array, you can use object initialization syntax and make this code shorter, see code below:
int[] numbers = new int[4] { 1, 2, 3, 4};
There are two types of array in C#:
- Single Dimension
- Multi Dimension
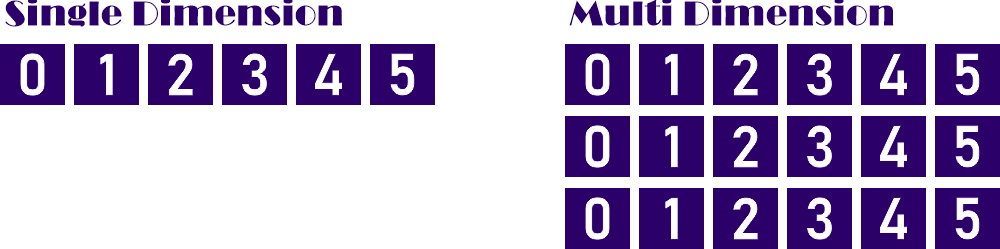
We have seen the example of single dimension above.
There are two types of Multi Dimensional arrays in C#:
- Rectangular
- Jagged
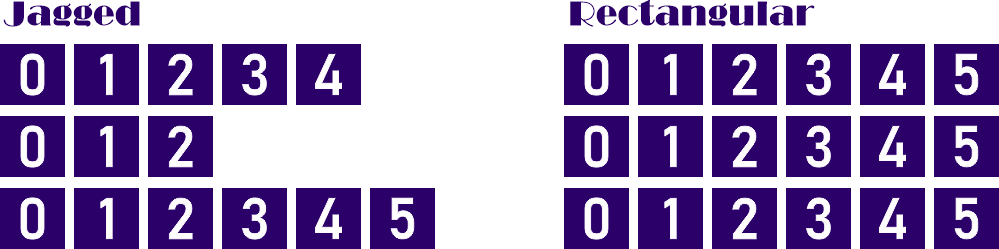
Rectangular Array:
In rectangular arrays each row has the same number of columns. In the example above we have 3 rows and each row has 6 columns. In jagged array the number of columns can be different. Below is how we declare a Rectangular array:
int[,] numbers = new int[4, 6];
In the above code, we have declared a rectangular array (2D) with 4 rows and 6 columns. We can also use var keyword instead of explicit type while declaring the variable.
var numbers = new int[4, 6];
If you hover over there var keyword in Visual Studio 2019, it will show it’s explicit type. c shar multi dimensional arrays
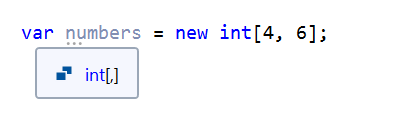
var numbers = new int[4, 6]
{
{1,2,3,4,5,6},
{7,8,9,10,11,12},
{13,14,15,16,17,18},
{19,20,21,22,23,24}
};
If we want to access the elements of these array, we will have to define row and column index. For example to access the number 16, we will have to tell compiler to get us the number from 3rd row and 4th column and it will be:
Console.WriteLine(numbers[2, 3]);
Since arrays are 0 indexed, 2 means the 3rd row and 3 means the 4th column.
If want to declare a 3 dimension array we do it the following way:
var numbers = new int[4, 6, 6];
Jagged Arrays:
In jagged array the length of column can be different for each row. To make it easier, think of Jagged array as an array of arrays.
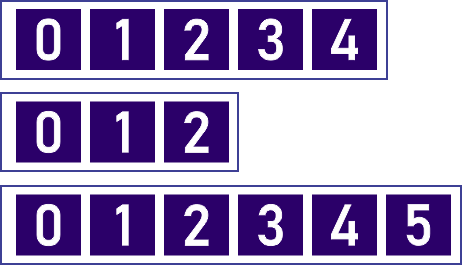
Top level has single dimension array of 5 elements, 2nd level has 3 elements and 3rd level has 6 elements.
array[0] = new int[5];
array[1] = new int[3];
array[2] = new int[6];
To declare a jagged array:
var jaggedArray = new int[3][];
Useful Array Methods:
int[] numbers = new int[4] { 1, 2, 3, 4};
Length:
Length method of array class returns the number of elements in an array. It returns zero if there are no elements in the array.
Console.WriteLine("Length of numbers Array " + numbers.Length); // 4
IndexOf :
IndexOf simply returns the index of the given value. If the given value is not in the array it returns -1. First parameter in that function is the array and second is the number whose index you are looking for.
var index = Array.IndexOf(numbers, 3);
Console.WriteLine(index); //2
Value 3 has the 3rd index but the IndexOf will return, because arrays are 0 indexed, first element has the index 0.
*** You might have noticed that we had to call IndexOf method with class name array. That is because IndexOf is a static method in Array class and can not be called with it’s instantiated objects.
Clear:
This method is used to clear certain elements in the array. If the array is an integer type, this function will make the element’s value to zero. If it is boolean, it will make it false and if it is string it will make it empty string.
When calling this method, the first parameter is the array, second parameter is the starting point meaning at which element clearance should start and 3rd parameter the number of elements it should continue till.
For example if we ant to clear the first two elements from our numbers array, we will write the following code:
Array.Clear(numbers, 0, 2);
So we are clearing the first 2 elements. We pass our array in the parameter, then we passed the first element where want to clearance to start and the 3rd parameter is the number of elements we should clear including the starting one. So as per our code it starts at 0 element and clears 2 elements including the element at 0 index.
If we go through our numbers array we will find the first two elements at 0.
for (int i = 0; i < numbers.Length; i++)
{
Console.WriteLine(numbers[i]);
}
/* Results
* 0
* 0
* 3
* 4
*/
Copy:
Let’s say we want to create another array with the first 3 number of our numbers array, we can use the copy method. This methods takes 3 parameters: Original array, Other array to copied to and number of elements to copy.
var otherArray = new int[3];
Array.Copy(numbers, otherArray, 3);
for (int i = 0; i < otherArray.Length; i++)
{
Console.WriteLine(otherArray[i]);
}
/* Results
* 1
* 2
* 3
*/
Sort:
This method sorts the elements in an array.
int[] unSortedArray = new int[10] { 10, 9, 8, 7, 6, 5, 4, 3, 2, 1 };
We can use the sort method to sort above array:
Array.Sort(unSortedArray);
for (int i = 0; i < unSortedArray.Length; i++)
{
Console.WriteLine(unSortedArray[i]);
}
//Result
/*
* 1
* 2
* 3
* 4
* 5
* 6
* 7
* 8
* 9
* 10
*/
Reverse:
Reverse simply does the opposite of sort method.