There comes a time in our program where we need data to represent Date and Time. In C# we use DateTime objects to represent date and time. Like int and double, DateTime is a structure of value type. It is availble in System namespace. If you look at the DateTime structure, you will find that it is a readonly.

For value types, readonly modifier makes sure that you can instantiate objects from the struct but it’s value can not be changed for the entire liftime of intance.
We can create a DateTime object using the new operator.
var dateTime = new DateTime(2015, 10, 22);
Constructor of DateTime structure has almost 11 overload constructors as seen in the image below:
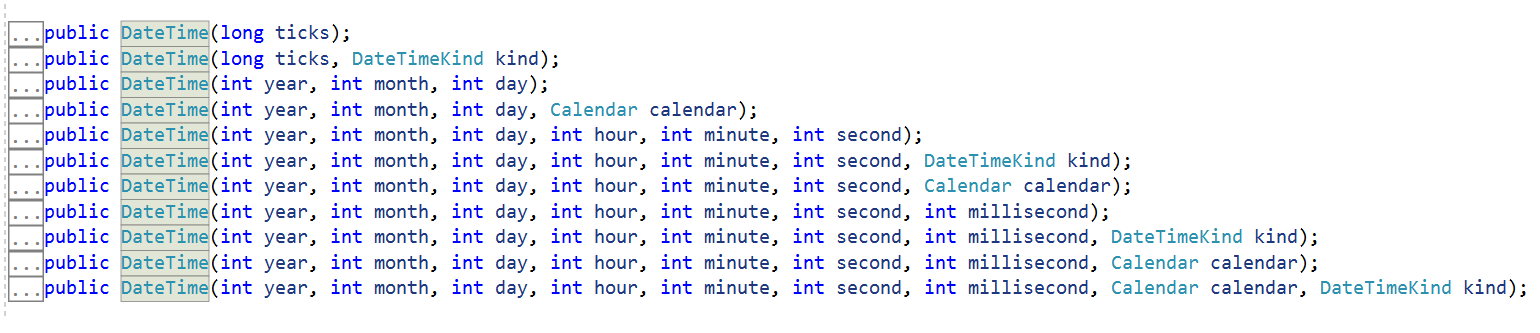
We will look at the few commonly used.
public DateTime (int year, int month, int day);
When you want to specify year, month and day in the instance of DateTime, we use this constructor.
var dateTime = new DateTime(2015, 10, 22);
Console.WriteLine(dateTime);
//outpute will be 2015-10-22 12:00:00 AM
When you specify instance of DateTime with this constructor, the time will always be midnight (00:00:00). Year, Month and Day has to be in the following range:
Year: Equal to or greater than 1 and equal to or less than 9999
Month: Equal to or greater than 1 and equal to or less than 12
Day: Equal to or greater than 1 and equal to or less than the days in that month.
DateTime(Int32, Int32, Int32, Calendar)
Output of the code above is the date of Gregorian Calender. If we wan’t to to get the dates of other calenders we would have to send that calender in DateTime Constructor.
var hijri = new HijriCalendar();
var todaysDate = new DateTime(1442, 05, 18, hijri);
Console.WriteLine(todaysDate);
// result will be
//2021-01-01 12:00:00 AM
We can see in the above results that date that we see in output is of Gregorian Calender. We can use the following methods to covert it to date of Hijri Calender.
Console.WriteLine(hijri.GetEra(todaysDate)); // 1
Console.WriteLine(hijri.GetYear(todaysDate)); // 1442
Console.WriteLine(hijri.GetMonth(todaysDate)); // 5
Console.WriteLine(hijri.GetDayOfMonth(todaysDate)); // 18
DateTime (int year, int month, int day, int hour, int minute, int second);
As we have noticed above that by default the time alwasy midnight but if we know the minute and second, we can pass it in the constructor.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59);
Console.WriteLine(dateTime); // 2020-12-31 11:59:59 PM
The above code we are getting the date one second before the 2021 stars. like year, month and day the 3 time parameters also have to be specific range:
hour: Equal to or greator than 1 and equal to or less then 23.
minute: Equal to or greator than 1 and equal to or less then 59
second: Equal to or greator than 1 and equal to or less then 59
DateTime (int year, int month, int day, int hour, int minute, int second, DateTimeKind kind);
To specify local time, Coordinated universal time also know as UTC or neither, we send DateTimeKind in the parameters of DateTime constructor. DateTimeKind is an enum in system namespace as shown below.
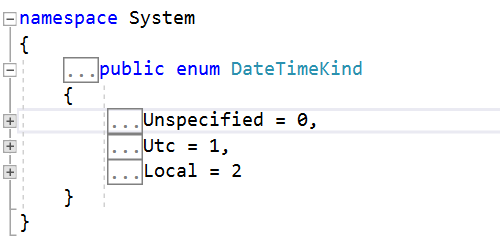
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, DateTimeKind.Local);
We can get the type of any DateTime object by using the Kind method.
Console.WriteLine(dateTime.Kind); // Local
DateTime (int year, int month, int day, int hour, int minute, int second, int millisecond);
We can also specify miliseconds in the DateTime constructor.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 500);
Useful DateTime Properties:
DateTime class also has multiple properties that we can use to get us to speed in our programe, let’s look at the few below.
DateTime.Now;
DateTime.Now method get’s you current date and time. It’s a static method so it has to be called with DateTime not it’s instantiated objects.
var currentDate = DateTime.Now;
Console.WriteLine(currentDate);
//results = 2021-01-02 6:27:09 PM
DateTime.Today;
DateTime.Today method get’s you today’s date and the time is midnight bye default. . It is also a static method so it has to be called with DateTime not it’s instantiated objects.
var todayDate = DateTime.Today;
Console.WriteLine(todayDate);
//results = 2021-01-02 12:00:00 AM
Year, Month, Day, Hour, Minute, Second, Millisecond
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
Console.WriteLine(dateTime); //Result = 2020-12-31 11:59:59 PM
//Let's get the Year from the date
Console.WriteLine("Year = " + dateTime.Year); //Year = 2020
//Let's get the Month from the date
Console.WriteLine("Month = " + dateTime.Month); //Month = 12
//Let's get the Day from the date
Console.WriteLine("Day = " + dateTime.Day); //Day =
//Let's get the Minute from the date
Console.WriteLine("Minute = " + dateTime.Minute); //Minute = 59
//Let's get the Second from the date
Console.WriteLine("Second = " + dateTime.Second); //Second = 59
//Let's get the Millisecond from the date
Console.WriteLine("Millisecond = " + dateTime.Millisecond); //Millisecond = 999
Useful DateTime Methods:
ToLongDateString()
As the name suggests, this method returns long date string representation of DateTime object. It only returns the date, not the time.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
Console.WriteLine(dateTime.ToLongDateString()); //Result = December 31, 2020
ToLongTimeString()
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
Console.WriteLine(dateTime.ToLongTimeString()); //Result = 11:59:59 PM
ToShortDateString ()
As oppose to LongDate, it returns short date string representation of DateTime Object.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
Console.WriteLine(dateTime.ToShortDateString()); //Result = 2020-12-31
ToShortTimeString ()
Returns short time string representation of DateTime Object. It returns time in hours and minutes.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
Console.WriteLine(dateTime.ToShortTimeString()); //Result = 11:59 PM
ToString()
This function coverts a DateTime object to it’s string representation.
var dateTimeToString = new DateTime(2020, 12, 31, 23, 59, 59, 999).ToString();
Console.WriteLine(dateTimeToString.GetType()); //Result = System.String
ToString(IFormatProvider)
Every country has a diffferent way to writing dates. We can overlaod the ToString method to get the date according to different countries. For this we can use the CultureInfo class. We won’t get into the details of CultureInfo at this moment. We can instansiate a CultureInfo object and use that to format the date for that culture.
var dateTime = new DateTime(2020, 12, 31, 23, 59, 59, 999);
var usCulture = new CultureInfo("en-us");
Console.WriteLine(dateTime.ToString(usCulture)); //Result = 12/31/2020 11:59:59 PM
Following code is for Canadian Date Format.
var CanadaCulture = new CultureInfo("en-CA");
Console.WriteLine(dateTime.ToString(CanadaCulture)); //Result = 2020-12-31 11:59:59 PM
We can also overload ToString method with out own format specifier.
Console.WriteLine(dateTime.ToString("yyyy-MM-dd")); //Result = 2020-12-31
We also specify time in it as well.
Console.WriteLine(dateTime.ToString("yyyy-MM-dd HH:mm")); //Result = 2020-12-31 23:59