Lists are similar to arrays as we use them to store number of objects of the same type. With arrays we need to know the size of it at the time of declaration but with Lists we don’t need that and we can also add more elements to a List dynamically.
var numbers = new List<int>();
List is generic type as indicated inside angle brackets in the code above. Inside the angle brackets we specify the generic parameter which specify what type of list it is. In the case of above code we are creating a list of integers.
List can be of any type, strings, characters or any non primitive types.
Just like arrays if we know the objects we would like to store in a list we can initialize our list with using Object Initialization syntax as you see below.
var numbers = new List<int>() {1, 2, 3, 4, 5};
Above code will give you an error on Microsoft Visual Studio 2019 as below:
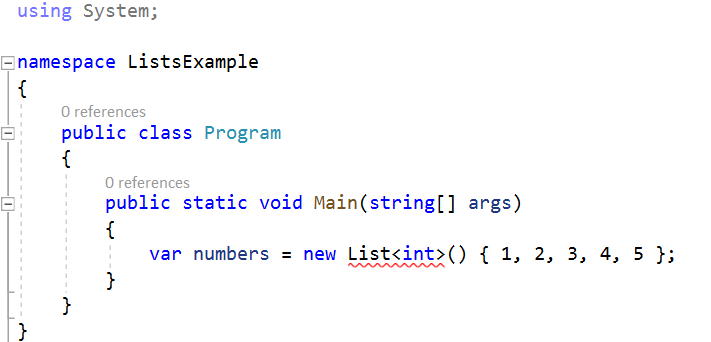
if you hover over the red lines, you will be able to read the error:

Since List is generic type so we have to use import Generic namespace.
using System.Collections.Generic;
Useful List Methods:
Add:
Unlike arrays, we can add elements to list by using Add method.
numbers.Add(1);
This will add a number 1 at the end of the list, let’s print our list and see the results.
foreach(var number in numbers)
{
Console.WriteLine(number);
}
/* Results
* 1
* 2
* 3
* 4
* 5
* 1
*/
AddRange:
AddRange method is used to add more then one numbers or other type. With AddRange we can add a collection of elements (array or list) at the end of the list. This function can reduce the use of foreach and for loops for an attempt to add more then one number in the list.
numbers.AddRange(new int[3] { 6, 7, 8 });
Above we added an array at the end of our numbers list and it will add 6,7,8 at the end of numbers list.
IndexOf:
You can provide an element to this method of a list and it will return it’s index.
var numbers = new List<int>() {1, 2, 3, 4, 5};
var index = numbers.IndexOf(5);
The above code will return 4, just like arrays, lists are also zero indexed.
LastIndexOf:
Let’s add another number into our list.
numbers.Add(3);
Now we will have two numbers in out list that are 3. The second number 3 will be added at the end of the list and when we use the method IndexOf it will return 2, the index of first 3 in the list. This is where we can use LastIndexOf method to get the last index of the number 3, see the code below.
var lastIndex = numbers.LastIndexOf(3); //5
The above code will return number 5. So if you want to start your search at the beginning of the list you use IndexOf method and if you want to start your search at the end of the list you use LastIndexOf method.
Count:
The Count method simply returns the number of objects in the list.
var numbers = new List<int>() {1, 2, 3, 4, 5};
var numbersOfObjects = numbers.Count;
Console.WriteLine(numbersOfObjects);
Above code will return 5 which is the number of objects in the numbers array.
Remove:
Remove removes the given element from the list.
var numbers = new List<int>() {1, 2, 3, 4, 5};
numbers.Remove(4);
The above code will remove number 4 from the list.